In this series of ASP.NET MVC Shopping Cart Application, Part-1 covered most of the administrative features including Category/Product Management and Project Initial Setup. Features we are about to cover in Part-2 for ASP.NET MVC Shopping Cart Application can be categorized in three major areas:
- User Registration and Authentication
- Register a New User
- Login User
- Product Display Center
- Displaying Feature Products
- Display Product by Category
- Product List View
- Single Product Detailed View
- Search Products
- Online Shopping Cart Management
- Add Products to Cart
- Cart View – List of Products added to Shopping Cart
- Remove Products from Shopping Cart
But along with that you need to prepare your practical skills on ASP.NET MVC technology.You can also find more related implementation details here:
- Take a FREE Online Test on ASP.NET MVC to verify you existing knowledge.
- Top New Features of ASP.NET vNext
- Building your first ASP.NET MVC 5 Application
- Understanding Model First Approach in MVC5 with EF6
- Understanding Application Life Cycle in ASP.NET MVC
- ASP.NET MVC3 Vs MVC4 Vs MVC5
- Pass Data from Controller to View (ViewBag | ViewData | TempData | Model)
User will land on home page by running the application. Below is the home page view.
ASP.NET MVC Shopping Cart Application homepage contains “Login” and “Registration” links on top right. Also, a user can search products by category using “Browse Categories” link and Searching Category/Products easily by name. Products those are marked as featured products are displayed in a slider view at the bottom of homepage.
Let’s start understanding the above mentioned features implementations using a step by step approach starting with User Registration and Authentication.
User Registration and Authentication:
User Registration is not a compulsory step for browsing the categories and products. But once the user add items to his/her Shopping Cart and wants to checkout, he/she will be redirected to “Login” page to authenticate. And if a user is not even registered, he/she will be moved to Sign Up for registration. User can also Sign Up by clicking on “SIGN UP” link at top right link.
Following is a simple and typical Sign Up screen with First Name, Last Name, Email Address and Password. User will provide these basic details and he/she will be registered with our Application.
A registered user can easily click on Login link, a Login popup will appear with Email as username and Password.
User puts his valid details and after submitting, he/she will be redirected accordingly with top right menu as welcome message, Cart Items and Logout links. The menu will be changed to:
Let’s go through the code For “Registration”. Add a controller named “AccountController” to our project having an Action method “Register” in it (detailed code as follows).
#region Member Registration ... [AllowAnonymous] public ActionResult Register() { return View(); } [HttpPost] [ValidateAntiForgeryToken] [ValidateInput(false)] public ActionResult Register(RegisterViewModel model, string returnUrl) { if (ModelState.IsValid) { // Adding Member Tbl_Members mem = new Tbl_Members(); mem.FirstName = model.FirstName; mem.LastName = model.LastName; mem.EmailId = model.UserEmailId; mem.Password = EncryptDecrypt.Encrypt(model.Password, true); mem.CreatedOn = DateTime.Now; mem.ModifiedOn = DateTime.Now; mem.IsActive = true; mem.IsDelete = false; _unitOfWork.GetRepositoryInstance<Tbl_Members>().Add(mem); // Adding Member Role Tbl_MemberRole mem_Role = new Tbl_MemberRole(); mem_Role.MemberId = mem.MemberId; mem_Role.RoleId = 2; _unitOfWork.GetRepositoryInstance<Tbl_MemberRole>().Add(mem_Role); TempData["VerificationLinlMsg"] = "You are registered successfully."; Session["MemberId"] = mem.MemberId; Response.Cookies["MemberName"].Value = mem.FirstName; Response.Cookies["MemberRole"].Value = "User"; return RedirectToAction("Index", "Home"); } return View("Register", model); } public JsonResult CheckEmailExist(string UserEmailId) { int LoginMemberId = Convert.ToInt32(Session["MemberId"]); var EmailExist = _unitOfWork.GetRepositoryInstance<Tbl_Members>().GetFirstOrDefaultByParameter(i => i.MemberId != LoginMemberId && i.EmailId == UserEmailId && i.IsDelete == false); return EmailExist == null ? Json(true, JsonRequestBehavior.AllowGet) : Json(false, JsonRequestBehavior.AllowGet); } #endregionAdd view of “Register” action with following code:
@model OnlineShopping.Models.RegisterViewModel @{ ViewBag.Title = "Register"; Layout = "~/Views/Shared/_FrontLayout.cshtml"; } <!--Start Sign Up--> <div class="contactUs signupBox clearfix"> <div class="container"> <h1>Sign Up</h1> @using (Html.BeginForm("Register", "Account", new { ReturnUrl = ViewBag.ReturnUrl }, FormMethod.Post, new { @class = "contactForm signupForm", role = "form" })) { @Html.AntiForgeryToken() <div class="signUpcheck clearfix" style="display:none"> <div class="customRadio checkRight"> @Html.RadioButton("UserType", 2, new { @class = "css-checkbox", @checked = "checked" }) <label for="radio-2" class="css-label">knowbee</label> </div> </div> <div class="formRow clearfix"> <div>@Html.ValidationMessageFor(m => m.FirstName) @Html.TextBoxFor(m => m.FirstName, new { @class = "inputComn", @placeholder = "First Name" })</div> </div> <div class="formRow clearfix"> <div> @Html.ValidationMessageFor(m => m.LastName) @Html.TextBoxFor(m => m.LastName, new { @class = "inputComn", @placeholder = "Last Name" })</div> </div> <div class="formRow clearfix"> <div> @Html.ValidationMessageFor(m => m.UserEmailId) @Html.TextBoxFor(m => m.UserEmailId, new { @class = "inputComn", @placeholder = "Email Address" })</div> </div> <div class="formRow clearfix"> <div> @Html.ValidationMessageFor(m => m.Password) @Html.PasswordFor(m => m.Password, new { @class = "inputComn", @placeholder = "Password", @type = "Password" })</div> </div> <div class="formRow clearfix"> <div> @Html.ValidationMessageFor(m => m.ConfirmPassword) @Html.PasswordFor(m => m.ConfirmPassword, new { @class = "inputComn", @placeholder = "ConfirmPassword", @type = "Password" })</div> </div> <input type="submit" class="contactBtn signupBtn" value="Submit" /> } </div> </div> <!--End Sign Up-->
User Login
Add partial view “_Login” in “Shared” folder as:
Add following code in it:
@model OnlineShopping.Models.LoginViewModel @using (Ajax.BeginForm("_Login", "Account", FormMethod.Post, new AjaxOptions { OnSuccess = "OnSuccessLoginPopup", OnFailure = "OnSuccessLoginPopup", UpdateTargetId = "loginpopup", InsertionMode = InsertionMode.Replace })) { @Html.AntiForgeryToken() <input type="hidden" name="returnUrl" id="returnUrl" /> <div class="loginBox" id="loginPoupForm"> <div class="closepop"><img src="/images/closebtn.png" alt=""></div> <h1><img src="/images/loginImg.png" alt="">Log In</h1> <h4 style="color:green;text-align:center">@TempData["EmailVarifiedMsg"]</h4> <div class="loginconentBox"> <div class="cstmText"> @Html.TextBoxFor(m => m.UserEmailId, new { @class = "inputmsg", @placeholder = "EmailAddress", @onkeypress = "return OnlyLegalCharacters();" }) @Html.ValidationMessageFor(m => m.UserEmailId) </div> <div class="cstmText"> @Html.TextBoxFor(m => m.Password, new { @class = "inputlock", @type = "Password", @placeholder = "Password", @onkeypress = "return OnlyLegalCharacters();" }) @Html.ValidationMessageFor(m => m.Password) </div> <div class="cstmText clearfix"> <div class="checkboxLeft"> @Html.CheckBoxFor(m => m.RememberMe, new { @class = "LoginCheckbox" }) RememberMe </div> <div class="checkboxRight"> <a href="/account/forgotpassword" class="forgotPass"> Forgot Password?</a> </div> </div> <div class="cstmText"> <input type="submit" class="loginBtn_Submit" value="Log In" /> </div> </div> </div> } <script> function InitializeCloseLoginPopup() { $(".closepop").click(function () { $("#loginpopup").fadeOut(400); $("body").removeClass("loghiddens"); $("#loginpopup").fadeOut(400); }); } function OnSuccessLoginPopup() { InitializeCloseLoginPopup(); if ('@ViewBag.redirectUrl' != '') { window.location.href = '@Html.Raw(ViewBag.redirectUrl)'; } } </script>
Add following code in “AccountController” to execute the login action:
#region Member Login ... [HttpPost] [ValidateAntiForgeryToken] public ActionResult _Login(LoginViewModel model, string returnUrl) { if (ModelState.IsValid) { string EncryptedPassword = EncryptDecrypt.Encrypt(model.Password, true); var user = _unitOfWork.GetRepositoryInstance<Tbl_Members>().GetFirstOrDefaultByParameter(i => i.EmailId == model.UserEmailId && i.Password == EncryptedPassword && i.IsDelete == false); if (user != null && user.IsActive == true) { Session["MemberId"] = user.MemberId; Response.Cookies["MemberName"].Value = user.FirstName; var roles = _unitOfWork.GetRepositoryInstance<Tbl_MemberRole>().GetFirstOrDefaultByParameter(i => i.MemberId == user.MemberId); if (roles != null && roles.RoleId != 3) { Response.Cookies["MemberRole"].Value = _unitOfWork.GetRepositoryInstance<Tbl_Roles>().GetFirstOrDefaultByParameter(i => i.RoleId == roles.RoleId).RoleName; } else { ModelState.AddModelError("Password", "Invalid username or password"); return View(model); } if (model.RememberMe) { Response.Cookies["RememberMe_UserEmailId"].Value = model.UserEmailId; Response.Cookies["RememberMe_Password"].Value = model.Password; } else { Response.Cookies["RememberMe_UserEmailId"].Expires = DateTime.Now.AddDays(-1); Response.Cookies["RememberMe_Password"].Expires = DateTime.Now.AddDays(-1); } ViewBag.redirectUrl = (!string.IsNullOrEmpty(returnUrl) ? HttpUtility.HtmlDecode(returnUrl) : "/"); } else { if (user != null && user.IsActive == false) ModelState.AddModelError("Password", "Your account in not verified"); else ModelState.AddModelError("Password", "Invalid username or password"); } } return PartialView("_Login", model); } #endregion
Below the search box, there is a slider of featured Products.
Product Display Center for ASP.NET MVC Shopping Cart
To create the home page, you need to create “HomeController” in controllers folder. Do it as follows:
Right click on controllers folder and click on add new controller.
It will create a Controller class as:
using OnlineShopping.Repository; using System.Linq; using System.Web.Mvc; using OnlineShopping.DAL; using OnlineShopping.Filters; namespace OnlineShopping.Controllers { public class HomeController : Controller { public ActionResult Index() { } } }Adding “HomePage” view
Add Index view by clicking on “Index” Action as:
add following code in the index view
@{ ViewBag.Title = "Online Shopping : Home Page"; Layout = "~/Views/Shared/_FrontLayout.cshtml"; List<OnlineShopping.DAL.Tbl_Product> FeaturedProducts = (List<OnlineShopping.DAL.Tbl_Product>)ViewBag.FeaturedProducts; } <style> .profPerson .ProfImg { width: 240px; height: 153px; } .assistImg .transition { height: 107px; } </style> <div class="homeSlider"> <div class="bannerSlider" style="background: url(../images/HomePageBanner1.jpg) no-repeat center center;"> </div> <div class="searchOnline clearfix"> <div class="container"> <div class="olContent fl"><h2>Search Product/Category</h2></div> <div class="olSearch fr"> @using (Html.BeginForm("Index", "Search", FormMethod.Post)) { <div><input type="text" placeholder="Enter Keywords" onkeypress="return onlynumericOrCharacter();" name="searchKey" class="inputComn houseText"></div> <div class="searchIcon"> <button type="button" class="searchBtn" onclick="$(this).parent().parent().submit();"><img src="/images/searchIcon.png" alt="img"></button> </div> } </div> </div> </div> </div> <!--End Slider--> <!--Start Featured Professionals--> <div class="featuredProfessionals"> <div class="container"> <h1>Featured Products</h1> <div class="professionSLider"> <div class="profSlider"> @foreach (var featured in FeaturedProducts) { <div class="item"> <div class="profPerson"> @{ if (File.Exists(Server.MapPath(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Medium/" + featured.ProductId + "_" + featured.ProductImage))) { <img class="ProfImg" title="Click to view product detail" style="cursor:pointer;" onclick="window.location.href ='/search/productdetail?pId=@featured.ProductId'" src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Medium/" + featured.ProductId+"_"+featured.ProductImage)" /> } else { <img class="ProfImg" title="Click to view product detail" style="cursor:pointer;" onclick="window.location.href ='/search/productdetail?pId=@featured.ProductId'" src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage_Default_Medium"])" alt="" /> } } <div class="profMid clearfix"> <h2 title="Click to view product detail" style="cursor:pointer;"><a href="/search/productdetail?pId=@featured.ProductId"> @featured.ProductName</a></h2> <div class="leftprof fl"><text>Category <a title="Products of this category" style="cursor:pointer;" href="/search?searchkey=@featured.Tbl_Category.CategoryName">@featured.Tbl_Category.CategoryName</a></text></div> </div> </div> <div class="profLast clearfix"> <div class="leftprofLast fl"><h4>Price</h4></div> <div class="rightprofLast fr"><h5>@(featured.Price)</h5></div> </div> </div> } </div> <div class="left-symbol"><img src="/Images/sliderRightarrow.png" alt="images"></div> <div class="right-symbol"><img src="/Images/sliderLeftarrow.png" alt="images"></div> </div> </div> </div> <!--End Featured Professionals--> @section Scripts{ <script> window.onload = function () { if ('@Request.QueryString["returnUrl"]' != '') { $('#returnUrl').val('@Request.QueryString["returnUrl"]') $('.loginpopup').trigger('click'); } if ('@(Request.UrlReferrer !=null ?Request.UrlReferrer.AbsolutePath.ToLower():"")' == '/account/resetpassword') { $('.loginpopup').trigger('click'); } if('@TempData["VerificationLinlMsg"]'!='') { swal('@TempData["VerificationLinlMsg"]', '', 'success'); } } </script> }Add the following code “Index” action:
// Instance on Unit of Work public GenericUnitOfWork _unitOfWork = new GenericUnitOfWork(); public ActionResult Index() { ViewBag.FeaturedProducts = _unitOfWork.GetRepositoryInstance<Tbl_Product>().GetListByParameter(i => i.IsFeatured == true).ToList(); return View(); }
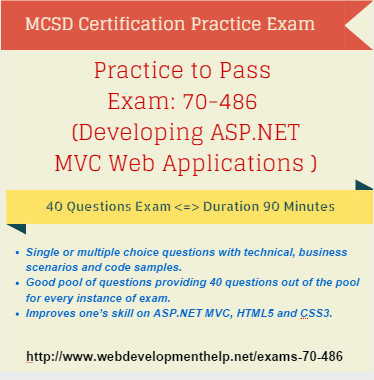
Search Section
Enter any product name or category name and click the search icon:
User can view the products per category by clicking on “Browse Category” button. Screen will appear as:
On click of category name or product name, the all the products matching the search key will appear.
The screen will look as:
Search Controller:
Add search controller and put following code in it. Search controller will look as:
using OnlineShopping.Repository; using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using OnlineShopping.DAL; using System.Data.SqlClient; using System.Data; using OnlineShopping.Filters; namespace OnlineShopping.Controllers { [FrontPageActionFilter] public class SearchController : Controller { #region Other Class references ... // Instance on Unit of Work public GenericUnitOfWork _unitOfWork = new GenericUnitOfWork(); #endregion /// <summary> /// Search result page /// </summary> /// <param name="searchKey"></param> /// <returns></returns> public ActionResult Index(string searchKey = "") { ViewBag.searchKey = searchKey; List<USP_Search_Result> sr = _unitOfWork.GetRepositoryInstance<USP_Search_Result>().GetResultBySqlProcedure ("USP_Search @searchKey", new SqlParameter("searchKey", SqlDbType.VarChar) { Value = searchKey }).ToList(); return View(sr); } } }Creating and Using Stored Procedure:
Create procedure “USP_Search” in database. following is the code to create a stored procedure in database:
Create proc [dbo].[USP_Search](@searchKey varchar(100)) as Begin select p.Description,p.Price,p.ProductId,p.ProductImage, p.ProductName, c.CategoryName from Tbl_Product p join Tbl_Category c on p.CategoryId=c.CategoryId where p.IsActive=1 and p.IsDelete=0 and c.IsActive=1 and c.IsDelete=0 and (p.ProductName like '%'+@searchKey+'%' or c.CategoryName like '%'+@searchKey+'%') End

Add following code in view:
@model IEnumerable<OnlineShopping.DAL.USP_Search_Result> @{ ViewBag.Title = "Search Result"; Layout = "~/Views/Shared/_FrontLayout.cshtml"; } <style> .servicesBox .servicesImg img { height: 172px; width: 270px; } .DservicesImg img { height: 162px; width: 254px; } .FilterBox { max-height: 200px; overflow: auto; } </style> @using (Html.BeginForm()) { <div id="dvFilterSearch"> <div class="mainHouse"> <div class="container"> <div class="housekeeping clearfix"> <div class="housekeepingLeft fl"><h1>@ViewBag.searchKey <span>@(!string.IsNullOrEmpty(ViewBag.searchKey) ? "I" : "") @(Model != null ? Model.Count() : 0) records found</span></h1> </div> <div class="housekeepingRight fr" id="divFilter"> <div class="housekeepingInput"><input type="text" placeholder="Enter Keywords" class="houseComn" id="searchKey" name="searchKey" value="@ViewBag.searchKey" onkeypress="return onlynumericOrCharacter();"></div> <div class="housekeepingSearch"><button type="button" class="housesearchBtn" onclick="$(this).parent().parent().submit();"><img src="/images/searchIcon.png" alt="img" /></button></div> </div> </div> </div> </div> <div class="mainServices clearfix"> <div class="container"> <!-- Start servicesRight--> <div class="servicesRight"> @if (Model.Count() > 0) { <!--Start pricelowHigh--> <div class="pricelowHigh clearfix"> <!--Start Services Man--> <div class="tabContent" id="tab1"> <div class="servicesPerson clearfix"> <ul class="prsonList"> @foreach (var item in Model) { <li> <!--Start ServicesBox--> <div class="servicesBox"> <div class="servicesImg"> @if (File.Exists(Server.MapPath(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "/Medium/" + item.ProductId + "_" + item.ProductImage))) { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage"]+"/Medium/"+item.ProductId+"_"+item.ProductImage)" alt="img"> } else { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage_Default"])" alt="img"> } </div> <div class="nameLink"> <a title="View Product" href="/search/productDetail?pId=@item.ProductId">@item.ProductName</a> <div class="byperson clearfix"> <h3>Category: @item.CategoryName</h3> </div> </div> <div class="rate clearfix"> <span class="fl">Price</span> <span class="blueClr fr">@item.Price</span> </div> </div> <!--End ServicesBox--> </li> if (((Model.ToList().IndexOf(item) + 1) % 4) == 0) { <div class="clearfix"></div> <br /> } } </ul> <!--End ServicesBox--> </div> <!--End Services Man--> </div> </div> } else { <div class="tabContent" id="tab3"> <!--Start Services Man--> <div class="clearfix"> <!--Start detailServicesBox--> <div class="detailservicesBox clearfix"> <div style="background-color: #fff; text-align: center; padding: 40% 0; "> <h2>No Records found</h2> </div> </div> </div> </div> } </div> </div> </div> <br /> <!--Start main services--> </div> } <!--End main services--> @section Scripts{ <script type="text/javascript"> $(document).ready(function () { if('@TempData["ProductAddedToCart"]'!='') { swal('@TempData["ProductAddedToCart"]', '', 'success'); } }); $('#searchKey').keydown(function (e) { if (e.keyCode == 13) { $('#dvFilterSearch').parent().submit(); } }) </script> }
Product Detail View:
Once the product is searched by category or by directly using the search product option, a user clicks on product to view the details about that specific item, he/she will be redirected to product detail page:
Here on Product detail page, user will be able to view all details about any specific application, it’s specification and price etc. He/She will be able to add item to Shopping Cart. MVC Shopping Cart code details will be covered later in this tutorial.
Create Action “ProductDetail” in “SearchController”. The code is:
/// <summary> /// Product Detail Page /// </summary> /// <param name="pId"></param> /// <returns></returns> public ActionResult ProductDetail(int pId) { Tbl_Product pd = _unitOfWork.GetRepositoryInstance<Tbl_Product>().GetFirstOrDefault(pId); ViewBag.SimilarProducts = _unitOfWork.GetRepositoryInstance<Tbl_Product>().GetListByParameter(i => i.CategoryId == pd.CategoryId).ToList(); return View(pd); }
Add following code in “ProductDetail” view:
@model OnlineShopping.DAL.Tbl_Product @using OnlineShopping.DAL @{ ViewBag.Title = "ServiceDetail"; Layout = "~/Views/Shared/_FrontLayout.cshtml"; List<Tbl_Product> SimilarProducts = (List<Tbl_Product>)ViewBag.SimilarProducts; } <style> .servicesList .servicesBox .servicesImg img { height: 145px; } </style> <!--Start product Details--> <div class="servicefinal"> <div class="container"> <div class="servicefinalLeft fl"> <!--Start servicesdetailsBox--> <div class="servicesdetailsBox"> @{ if (File.Exists(Server.MapPath(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Large/" +Model.ProductId+ "_" + Model.ProductImage))) { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Large/" +Model.ProductId+ "_" + Model.ProductImage)" /> } else { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage_Default_Large"])" alt="" /> } } <div class="inrfinalServices"> <h2>@Model.ProductName</h2> <h3>@Model.Tbl_Category.CategoryName</h3> </div> </div> <!--End servicesdetailsBox--> <!--Start Description--> <div class="description"> <!--Start aboutMe--> <div class="aboutMe"> <h1 class="prfHeading">Description</h1> <div class="profInnerBox innerbox1"> @Html.Raw(Model.Description) </div> </div> <!--End aboutMe--> </div> <!--End Description--> </div> <div class="servicefinalRight fr"> <!--Start Add product to cart--> <div class="bookaService"> <span>Price</span> <strong>@Model.Price</strong> <a href="/shopping/addproducttocart?productId=@Model.ProductId" class="bookreviewBtn" type="button">Add to cart</a> </div> <!--End Add product to cart--> <!--Start Similar Products--> <div class="favorite"> <div class="othersViewed"><span>Similar Products</span></div> </div> <div class="viewList"> <ul class="servicesList"> @if (SimilarProducts.Count > 0) { foreach (var ov in SimilarProducts) { <li> <!--Start ServicesBox--> <div class="servicesBox"> <div class="servicesImg"> @if (File.Exists(Server.MapPath(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Medium/" + ov.ProductId + "_" + ov.ProductImage))) { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "Medium/" + ov.ProductId + "_" + ov.ProductImage)" /> } else { <img src="/images/No Photo.png" alt="" />} </div> <div class="nameLink"> <a href="/search/servicedetproductdetail?pId=@ov.ProductId" title="View product details">@ov.ProductName</a> <div class="byperson clearfix"> <div class="prsonLeft fl"><h3>Category : @ov.Tbl_Category.CategoryName</h3></div> </div> </div> <div class="rate clearfix"> <span class="fl">Price</span> <span class="blueClr fr">@ov.Price</span> </div> </div> <!--End Similar Products--> </li> } } else { <li> No similar products <div class="servicesBox"></div> </li> } </ul> <!--End list--> </div> </div> </div> </div> <!--End product Details-->
- Learn ASP NET MVC 5 step by step [Maruti Makwana, Corporate Trainer]
28 Lectures, 2.5 Hours Video, Intermediate Level
Very easy to learn video series on Asp.Net MVC 5 Specially for those who are familiar with Asp.Net Web forms. - AngularJS for ASP.NET MVC Developers [Brett Romero]
10 Lectures, 1 hour video, Intermediate Level
The Fastest Way For .NET Developers To Add AngularJS To Their Resume - ASP.NET with Entity Framework from Scratch
[Manzoor Ahmad, MCPD | MCT] 77 Lectures, 10 hour video, All Level
Latest approach of web application development - Comprehensive ASP.NET MVC [3D BUZZ]
34 lectures, 14 Hours Video, All Levels
From zero knowledge of ASP.NET to deploying a complete project to production.
Online Shopping Cart Management:
Add Product to cart:
Click on “Add to Cart” button to add product to cart.
If user is not login, he will be redirected to login, else the product will be added to his cart and user will get the successful message as shown below:
To manage the products in cart, we need to create a table Tbl_Cart. The table structure is:
There is an another table Tbl_CartStatus that has the Cart item status.
Add an action in Shopping controller to add product to cart.
The code is:
/// <summary> /// Add Product To Cart /// </summary> /// <param name="productId"></param> /// <returns></returns> public ActionResult AddProductToCart(int productId) { Tbl_Cart c = new Tbl_Cart(); c.AddedOn = DateTime.Now; c.CartStatusId = 1; c.MemberId = memberId; c.ProductId = productId; c.UpdatedOn = DateTime.Now; _unitOfWork.GetRepositoryInstance<Tbl_Cart>().Add(c); _unitOfWork.SaveChanges(); TempData["ProductAddedToCart"] = "Product added to cart successfully"; return RedirectToAction("Index", "Search"); }
View Items in Cart
User can see the items in his cart by clicking on the “Cart Items”. The page will list the products in the cart.
The page will look like:
To list the items, create an action “MyCart” in Shopping controller. It will contain following code:
public ActionResult MyCart() { List<USP_MemberShoppingCartDetails_Result> cd = _unitOfWork.GetRepositoryInstance<USP_MemberShoppingCartDetails_Result>() .GetResultBySqlProcedure("USP_MemberShoppingCartDetails @memberId", new SqlParameter("memberId", System.Data.SqlDbType.Int) { Value = memberId }).ToList(); return View(cd); }
The data is fetched from database using stored procedure. The procedure is:
create proc USP_MemberShoppingCartDetails (@memberId int) as Begin select p.Price,p.ProductId,p.ProductImage,p.ProductName,c.CategoryName from Tbl_Cart cr join Tbl_Product p on p.ProductId=cr.ProductId join Tbl_Category c on c.CategoryId=p.CategoryId join Tbl_Members m on m.MemberId=cr.MemberId where m.MemberId=@memberId and cr.CartStatusId=1 End
View will contain following code:
@model List<OnlineShopping.DAL.USP_MemberShoppingCartDetails_Result> @{ ViewBag.Title = "MyCart"; Layout = "~/Views/Shared/_FrontLayout.cshtml"; } <style> .detailLeft { width: 20% !important; position: relative; } .detailRight { width: 80%; } .DservicesImg img { width: 140px; } .FilterBox { max-height: 200px; overflow: auto; } .RemoveCartBtn { background-color: #005faa; color: white; padding: 10px 0; width: 100%; cursor: pointer; max-width: 160px; margin: 22px; display: table; border: 1px solid transparent; text-align: center; text-decoration: none; font-family: 'Open Sans'; text-transform: capitalize; position: absolute; right: 0; top: 0; } .innerDetails { height: auto !important; position: relative; } .detailservicesBox{border:none !important;} </style> <div id="dvFilterSearch" style="margin-top:30px;"> <div class="mainServices clearfix"> <div class="container"> <h2 style="margin:20px;text-align:center;font-weight:bold;">My Cart Details</h2> <!-- Start servicesRight--> <div class="servicesRight"> @if (Model.Count() > 0) { <!--Start pricelowHigh--> <div class="pricelowHigh clearfix"> <!--Start Services Man--> <div class="tabContent" id="tab2"> <!--Start Services Man--> <div class="servicesPerson clearfix"> @foreach (var item in Model) { <!--Start detailServicesBox--> <div class="detailservicesBox clearfix"> <div class="detailLeft fl"> <div class="DservicesImg"> @if (File.Exists(Server.MapPath(System.Configuration.ConfigurationManager.AppSettings["ProductImage"] + "/Medium/" + item.ProductId + "_" + item.ProductImage))) { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage"]+"/Medium/"+item.ProductId+"_"+item.ProductImage)" alt="img" /> } else { <img src="@(System.Configuration.ConfigurationManager.AppSettings["ProductImage_Default"])" alt="img" /> } </div> </div> <div class="detailRight fr"> <div class="innerDetails"> <h1><a title="View Product Detail" href="/search/ProductDetail?pId=@item.ProductId">@item.ProductName</a></h1> <h3>Category <a style="cursor:pointer" title="Click to View the products of this category" href="/search/knowbleprofile?pId=@item.ProductId">@(item.ProductName)</a></h3> <p><b>Price : </b><b>@item.Price</b></p> <p><a href="/shopping/removecartitem?productId=@item.ProductId" onclick="show_progress();" class="RemoveCartBtn">Remove from cart</a></p> </div> </div> </div> } </div> </div> </div> } else { <div class="tabContent" id="tab3"> <div class="clearfix"> <div class="detailservicesBox clearfix"> <div style="background-color: #fff; text-align: center; padding: 40% 0; "> <h2>No Records found</h2> </div> </div> </div> </div> } </div> </div> </div> </div>
Remove Product From Shopping Cart
User can remove an item from cart by clicking on the button “Remove From Cart” and that item will be remove from the cart.
Following code is done to complete this action:
/// <summary> /// Remove Cart Item /// </summary> /// <param name="productId"></param> /// <returns></returns> public ActionResult RemoveCartItem(int productId) { Tbl_Cart c = _unitOfWork.GetRepositoryInstance<Tbl_Cart>().GetFirstOrDefaultByParameter(i => i.ProductId == productId && i.MemberId == memberId && i.CartStatusId == 1); c.CartStatusId = 2; c.UpdatedOn = DateTime.Now; _unitOfWork.GetRepositoryInstance<Tbl_Cart>().Update(c); _unitOfWork.SaveChanges(); return RedirectToAction("MyCart"); }
Finally, we are able to complete major part of our Online Shopping Cart Application in ASP.NET MVC with C#, Entity Framework and SQL Server. Remaining is more related to Order Placement and Order Management in Administrative Area that will be covered in Part-3.
Part-1 – Building ASP.NET MVC Online Shopping Cart Application
Other Related Articles:
- 3 simple steps to create your first ASP.NET Web API Service
- Performing All CRUD Operations Using ASP.NET Web API
- Model Validation in ASP.NET Web API
- Exception Handling in ASP.NET Web API
- Understanding All About Controllers and Action Methods in ASP.NET MVC
Top Web Developer Interview Questions and Answers Series:
- Top 20 AngularJS Interview Questions
- Top 15 Bootstrap Interview Questions
- Top 10 HTML5 Interview Questions
- Top 10 ASP.NET Interview Questions
- Comprehensive Series of ASP.NET Interview Questions
- Top 10 ASP.NET MVC Interview Questions
- Top 10 ASP.NET Web API Interview Questions
- Top 10 ASP.NET AJAX Interview Questions
- Top 10 WCF Interview Questions
- Comprehensive Series of WCF Interview Questions
The post ASP.NET MVC Shopping Cart with C#, EF, SQL Server-Part2 appeared first on Web Development Tutorial.